Javascript is the most used language for web developement and it’s the language for browsers, you can do lot of things in javascript other than web scripting like building games, backend servers and what not. So the need for shuffling an array using javascript can arise for number of applications to randomize some behaviour or create new array from existing array etc. In this tutorials we will see multiple ways to shuffle array javascript using modern Javascript that is ES6 or greater. so let’s see how can we randomize an array javascript.
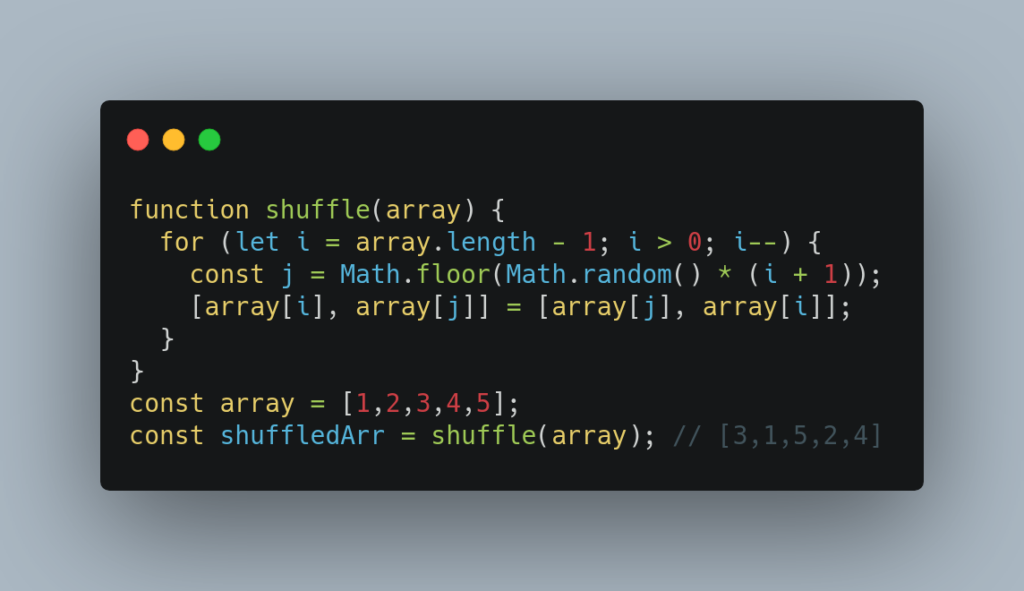
Article Contents
Shuffle Array Javascript using Array.map and Math.random
// using Array map and Math.random const shuffledArr = array => array.map(a => ({ sort: Math.random(), value: a })).sort((a, b) => a.sort - b.sort).map(a => a.value);
Shuffle Array Javascript using Array.sort and Math.random
// using Array sort and Math.random const shuffledArr = array => array.sort(() => 0.5 - Math.random());
Shuffle Array Javascript using Lodash shuffle method
// import or load lodash const shuffledArr = _.shuffle([1, 4,6,7,10]);
Shuffle an Javascript array using Fisher-Yates (aka Knuth) shuffle algorithm
function shuffle(array) { var currentIndex = array.length, temporaryValue, randomIndex; // While there remain elements to shuffle... while (0 !== currentIndex) { // Pick a remaining element... randomIndex = Math.floor(Math.random() * currentIndex); currentIndex -= 1; // And swap it with the current element. temporaryValue = array[currentIndex]; array[currentIndex] = array[randomIndex]; array[randomIndex] = temporaryValue; } return array; } const shuffledArr = shuffle(array);
Shuffle or randomize array using Durstenfeld shuffle algorithm
it is an optimized version of Fisher-Yates shuffle algorithm
function shuffle(array) { for (let i = array.length - 1; i > 0; i--) { const j = Math.floor(Math.random() * (i + 1)); [array[i], array[j]] = [array[j], array[i]]; } } const shuffledArr = shuffle(array);
So we have listed 5 ways to shuffle array javascript using multiple algorithms but recommended ones are Durstenfeld shuffle algorithm or Fisher-Yates (aka Knuth) shuffle. Durstenfeld shuffle algorithm is slighty faster compared to Knuth shuffle algorithm. It’s upto you to use any one of these methods to randomiz an array based on your requirement.
Resources:
- https://stackoverflow.com/questions/2450954/how-to-randomize-shuffle-a-javascript-array
- https://en.wikipedia.org/wiki/Fisher%E2%80%93Yates_shuffle#The_modern_algorithm
- https://1loc.dev/#shuffle-an-array