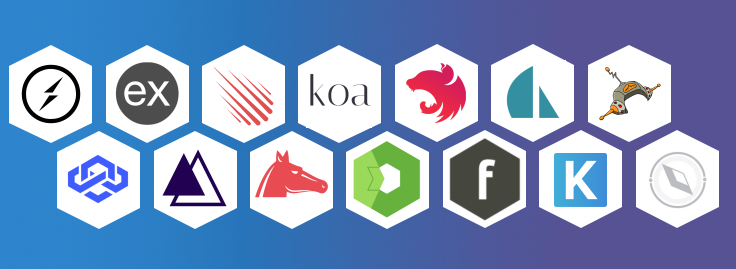
If you’re developing a small node script based on global node_module that you have installed, Once you import or require that particular node_module, You will see that Node.JS runtime will throw error module not found error, It is basically because your Node app doesn’t have access to globally installed node_modules, but thanks to vibrant Node.js community, we have found workarounds, we will see them below:
Article Contents
Require Global Node_Module in Node app using NODE_PATH ENV var
export NODE_PATH=$(npm root -g) or NODE_PATH=$(npm root -g) node index.js or in package.json file scripts "start":"NODE_PATH=$(npm root -g) node index.js"
NodeJS require a global module/package
const { execSync } = require("child_process"); // get root folder of global node modules const root = execSync("npm root -g") .toString() .trim(); // then we require global node modules as const axios = require(`${root}/axios`); const uuidv4 = require(`${root}/uuid/v4`);
Use global Node_Modules in Nodejs app using requireg npm module
It support both npm/yarn global packages installation paths.But you have to install it in your node app to use it.
const requireg = require('requireg'); // require a globally installed package const axios = requireg('axios'); // require a globally installed package and skip local packages const eslint = requireg('eslint', true);
Conclusion
You can use any of the above method, But second option is most preferrable, as it works without any third party node module or without require any env variable.